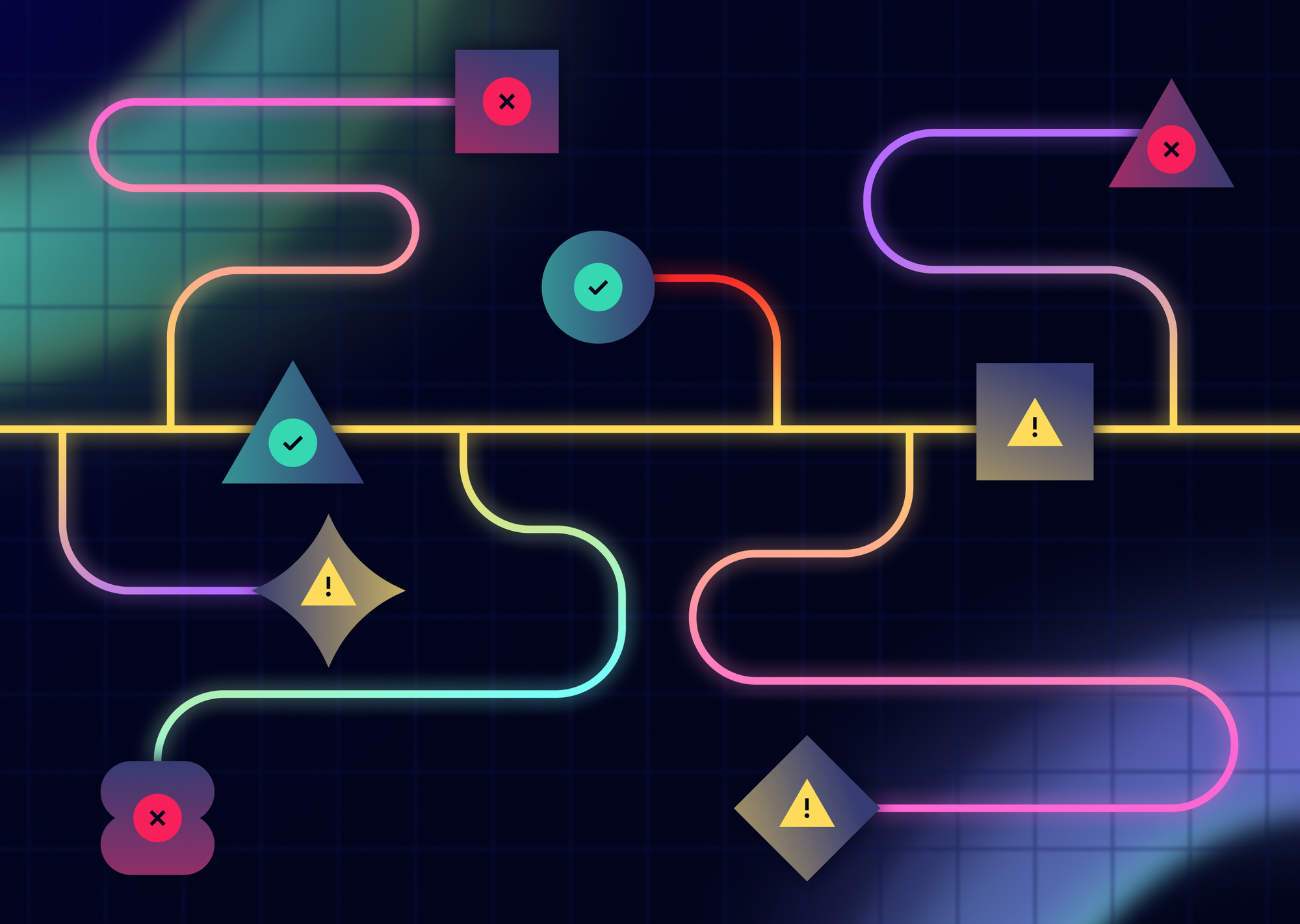
A software application typically consists of multiple small modules or components, each responsible for a specific functionality, such as user authentication, data retrieval, or form submission. A defect in one of these modules can render the entire application inoperable.
In this article, I’ll explain component testing, its objectives, types, techniques, and stages, and answer frequently asked questions.
What is component testing?
Component testing, also known as module testing, is a key practice in software testing that focuses on verifying individual components or units (such as functions, classes, or modules) in isolation before they are integrated into a complete system. It ensures that each component functions according to design specifications and helps us better understand the role each module plays and how it behaves under different conditions.
Testing each module separately aims to detect defects early, ensuring that every component performs as expected in various scenarios. This approach isolates potential issues and minimizes the risk of defects spreading throughout the system during integration. Thorough component testing helps confirm that each part of the software is reliable and ready for the integration phase.
Typically, component testing is performed after code implementation and unit testing but before integration testing to ensure that individual parts of a system function correctly before being combined.
Objectives of component testing
The primary goal of component testing is to isolate individual components and ensure that each one is defect-free, functions as intended, and meets specified requirements. Isolation in this context helps eliminate interference from other modules.
Key objectives of component testing
- Early defect detection: Identifying potential defects at an early stage of development.
- Requirement validation: Verifying compliance with both functional and non-functional requirements. Functional requirements define what the component should do, while non-functional requirements cover aspects such as performance, security, and scalability.
- Performance verification: Assessing the component’s efficiency, speed, and responsiveness.
- Reliability assurance: Ensuring that the component operates consistently over time, even under stress or error conditions.
- Facilitating integration: Preparing the component for seamless integration with other system modules. By testing each module independently, you can ensure that each one functions as expected, minimizing integration risks.
Component testing techniques
Component testing can be performed using two main approaches: Component Testing in Small (CTIS) and Component Testing in Large (CTIL). These methods help detect issues at both the individual module level and in their interactions.
Component Testing in Small (CTIS)
CTIS is conducted in isolation, without dependencies on other system components.
- Focuses on testing functions, methods, and classes
- Verifies how an individual component processes inputs and generates outputs
- Helps localize defects, making them easier to fix
- Frequently used in the early stages of development
CTIS is used to validate the logic of individual functions, test standalone classes or libraries, and debug without dependencies on other system parts.
Component Testing in Large (CTIL)
CTIL is performed in the context of other components when one module depends on another.
- Focuses on verifying integration and interactions between components
- Helps detect data transfer errors, interface inconsistencies, and compatibility issues
- Can use stubs and drivers to simulate missing components
- Ensures functionality in a real-world environment
CTIL tests API interactions or microservices, validates modules that depend on external services, and assesses system stability during data exchange.
Types of component testing
Component testing includes several types, each focusing on verifying specific aspects of component functionality and interaction.
Unit testing
Developers use unit testing during coding or before release. This type of testing examines individual modules or components of an application. Its goal is to ensure that each function, method, or class operates correctly in an isolated environment. Unit testing is useful for quickly detecting bugs in the early stages of development and simplifying debugging by testing small code units. Unit component testing is typically automated and runs frequently to maintain code stability.
Integration Testing
Integration testing verifies the interaction between multiple system components to ensure that modules work correctly together. It helps identify integration issues such as data transfer errors or API incompatibilities. This type of testing can be performed automatically or manually, covering scenarios that unit tests cannot, such as database and server-side logic interactions.
Interface testing
Interface testing focuses on verifying interaction points between software modules to ensure correct data exchange between components. It tests APIs, web services, databases, and front-end to back-end communication, guaranteeing that data is transmitted and processed without loss or errors. This testing also includes data format validation, such as JSON and XML, along with authentication and error handling.
Component interface testing
This type of testing checks the interaction of individual components through their interfaces, ensuring that each component correctly processes input and output data. It can be performed in isolation or as part of integration testing, evaluating component resilience under various input conditions. This approach is particularly useful for testing modular libraries, SDKs, and plugins.
Component testing strategies
When testing components, two primary approaches are commonly used: white-box testing and black-box testing. Their goal is to ensure that each component behaves as expected, identify potential defects, and verify compliance with requirements.
White-box testing
In white-box testing, the tester has access to the internal structure of the component and its implementation details. The tester analyzes the code, logic, and data flow to design test cases. This approach allows for thorough validation of all possible scenarios, including:
- Boundary conditions: testing minimum and maximum variable values
- Code branching: verifying all if, else, and switch conditions
- Error handling: checking cases such as division by zero or exception handling
Black-box testing
In black-box testing, the tester does not see the component’s code and has no knowledge of its internal workings. The focus is solely on input data, expected output values, and component behavior. This approach helps evaluate whether functionality meets requirements, including:
- Input validation: ensuring the system correctly handles valid and invalid inputs
- Functional correctness: verifying that the component produces expected outputs based on given inputs
- Error messaging: checking that the system provides appropriate feedback for incorrect or unexpected user actions
Phases of component testing
The component testing process consists of eight key phases:
1. Requirement analysis
Testers review functional and system specifications for each component, identifying key functions, constraints, and potential risks that may impact testing.
2. Test strategy
A comprehensive testing plan is developed, including the choice of approaches (manual or automated testing), tools, metrics, and exit criteria. The testability of requirements is also assessed.
3. Test specification
Test scenarios and test cases are defined. Critical tests are prioritized, while lower-priority tests may be deferred or omitted.
4. Test implementation
At this stage, test data is created, test cases are automated (if applicable), and the test environment is prepared.
5. Test execution
Test cases are executed to verify component functionality. Detected defects are logged in a bug-tracking system.
6. Test recording
Testing results are documented, including passed and failed tests, identified defects, and error reproduction steps. This data is used for further analysis.
7. Test verification
The quality of testing is assessed by comparing actual results with expected outcomes, evaluating test coverage, and analyzing detected defects.
8. Test closure
Final testing results are summarized, and components are evaluated for compliance with requirements. A final report is generated, and if necessary, a retrospective review is conducted to improve future testing processes.
Component testing examples
Example 1: Testing a login form
Scenario
The LoginForm component handles username and password input and submits credentials to the server.
What is tested?
Positive scenario
- Enter valid credentials
- Click the “Login” button
- Expect a successful login (redirect to the homepage, profile displayed)
Negative scenarios
- Enter incorrect username/password → error message appears
- Leave fields empty → validation message appears
- Enter a password shorter than six characters → validation error
Boundary values
- Enter 255 characters in the username field (maximum allowed)
- Enter only spaces as a password → The system should handle this correctly
UI tests
- Ensure the “Login” button is enabled only when fields are filled
- Verify the display of error messages
Example 2: Testing a product filtering component
Scenario
The ProductFilter component filters products in an online store by price, category, and availability.
What is tested?
Filter functionality
- Selecting the “Laptops” category → only laptops should be displayed
- Filtering by price ($50 - $100) → only products within this range appear
- Applying multiple filters (“Laptops” + “In Stock”) → only products matching both criteria should be shown
Boundary values
- Setting the minimum price higher than the maximum → the system should handle this correctly
- Filtering with no matching results → a “No items found” message should appear
Performance
- Verify filter response time when handling 10,000+ products
UI tests
- Ensure correct display of selected filters
- Verify the “Reset Filters” button design and behavior after clearing filters
FAQs about component testing
What is a test component?
A component is an independent module or a group of related functions in software. It is the smallest testable part of an application, which may include code, data structures, and interfaces. Component testing ensures that each unit performs as expected before integration.
Who is responsible for component testing?
Developers or dedicated testers typically perform component testing. Developers often conduct initial testing on their own code before handing it over for further validation by QA engineers.
What is the difference between API tests and component tests?
API testing focuses on verifying the external interfaces of one or multiple components. It checks data exchange, security, and performance between services.
Component testing ensures the internal functionality of a single component, often in isolation. It validates business logic, input/output processing, and error handling before integration.
How are stubs and drivers used in component testing?
A driver simulates a higher-level module that calls the component under test. It is used when the component does not yet have an actual calling module.
A stub acts as a placeholder for a lower-level component that is not yet implemented or available. It mimics responses to allow isolated testing of the component.
Stubs and drivers are commonly used in bottom-up and top-down integration testing strategies. They enable testing components independently before the entire system is assembled.
How can test automation be used for component testing?
Test automation enhances efficiency and accuracy in component testing by reducing manual effort. It can be applied in both white-box and black-box testing.
In white box testing, automated tools execute test cases based on internal structures, code coverage, and logic flows. In black-box testing, automation simulates user interactions and verifies expected outputs. Automated component testing is essential for continuous integration (CI/CD) pipelines, ensuring quick feedback and preventing regressions.