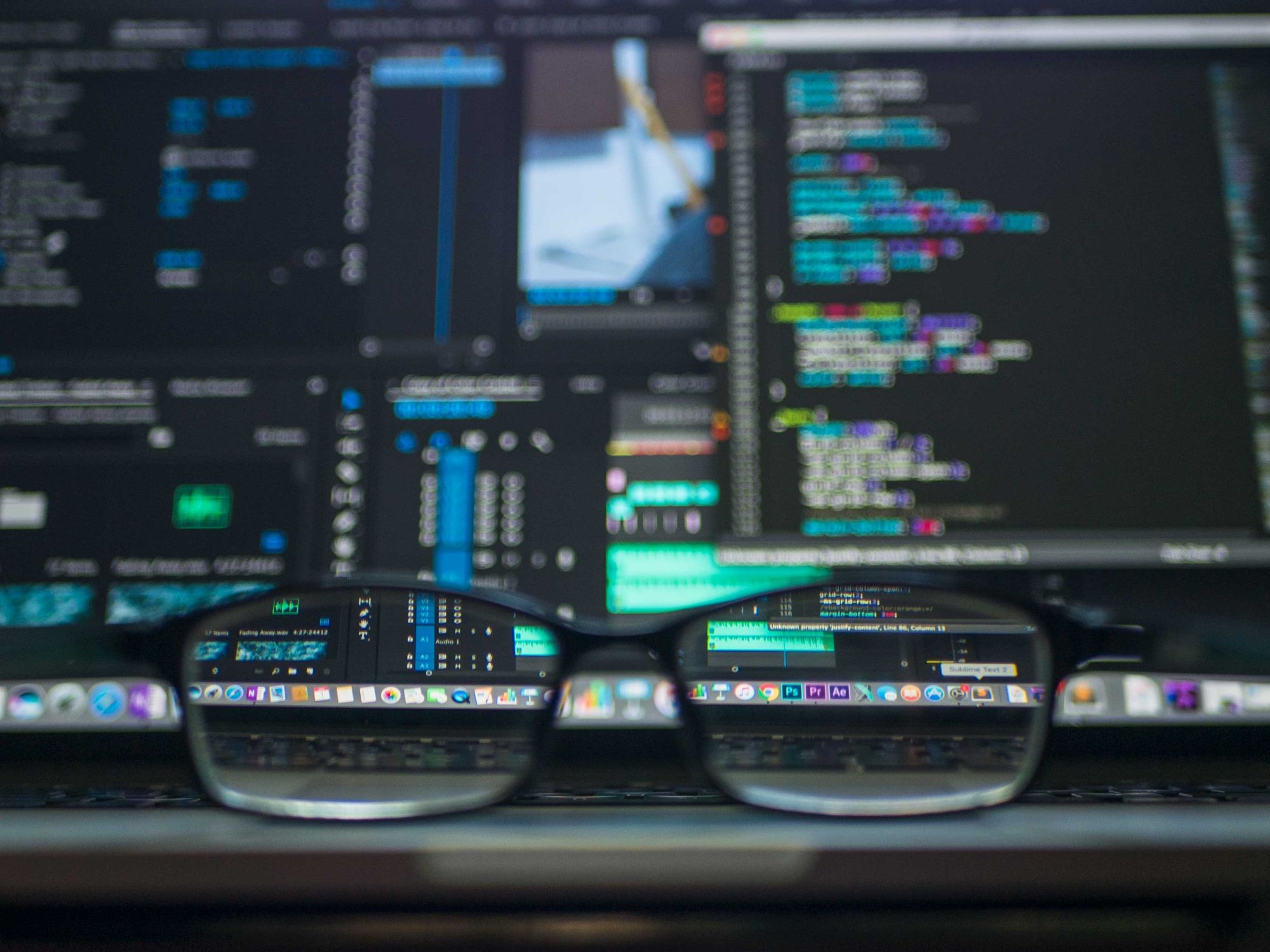
What is test driven development (TDD)? In short — TDD is a software testing approach that aims to create reliable code by testing the program before you write it.
Software developers are continually seeking ways to enhance the quality and efficiency of their work. One of the key methods to achieve these objectives is through tests. Automated tests play a pivotal role in software development, providing a systematic means to verify that the software functions as intended. These tests are written once and can be executed repeatedly, ensuring consistent and reliable results.
This article delves into the concept of test-driven development (TDD) by defining its principles, discussing its three fundamental laws, explaining the red-green-refactor cycle, and providing examples that highlight the benefits of TDD.
What is test driven development?
TDD is a software development process where test cases are created to define the desired behavior of the software. These test cases are formulated based on the software requirements before writing the actual code. Once the tests are in place, the code is then implemented to pass these tests and meet the specified requirements. This iterative approach ensures that the code is written to fulfill the intended functionality as defined by the tests.
Software engineer Kent Beck is credited with proposing this approach. He himself claims that he only rediscovered the method that existed at the dawn of programming and was described in the book “Digital Computer Programming” by D.D. McCracken, published in 1957.
TDD emerged as a fundamental practice within extreme programming (XP) and has become one of its most influential components. XP places significant emphasis on testing as a means to ensure software reliability and quality. It promotes incremental development — building software in small, manageable increments.
TDD methodology is also founded on the principles of the Agile manifesto. Agile development thrives on regular feedback to deliver the expected product incrementally, fostering close collaboration with stakeholders. TDD complements Agile by providing a disciplined and feedback-driven approach to software development. It enhances communication between developers, QA teams, and customers.
Robert C. Martin, an author of books on programming, also known as Uncle Bob and a co-author of the Agile Manifesto, formulated the Three laws of TDD:
- You are not allowed to write any production code unless it is to make a failing unit test pass.
- You are not allowed to write any more of a unit test than is sufficient to fail; and compilation failures are failures.
- You are not allowed to write any more production code than is sufficient to pass the one failing unit test.
In essence, the first law requires starting with a test that describes the desired functionality. The second law restricts the number of tests, and the third law mandates writing only enough code to pass the test. This approach ensures a precise balance between tests and code, resulting in a well-functioning system.
“One of the primary reasons I switched to TDD is for improved test coverage, which leads to 40%-80% fewer bugs in production. This is my favorite benefit of TDD. It’s like a giant weight lifting off your shoulders.” - Eric Elliott, software engineer, editor of JavaScript Scene and The Challenge.
Adhering to these laws allows us to strike the necessary balance between tests and code, making the development process more manageable. The requirement of writing tests one at a time enables a focused approach to each piece of code. On the contrary, ignoring these rules and adding numerous tests simultaneously can lead to code complexity and decreased quality.
One of the primary distinctions of TDD from traditional software development methods is its cyclical process, in contrast to the linear approach followed by the latter.
Red-green-refactor development cycle
The TDD cycle consists of three main phases: red, green, and refactoring. Red/Green/Refactor is the TDD mantra.
Red
Before adding a new feature, you write a test that describes the specifications the new feature should meet. You write only one simple test that is expected to fail. If it passes before lines of code are written, it means that the test is flawed.
It is essential to determine the extent of the code that the test will cover. The advantage of the TDD approach is that it allows you to make this decision based on your experience. If you are confident in what you are doing, you can cover a larger amount of code.
Kent Beck has emphasized many times that one of the most significant advantages of TDD is its ability to work in very small steps. With TDD, you can start with a large step but easily transition to smaller steps when difficulties arise.
“If a test method is getting long and complicated, then you need to play 'Baby Steps.' The goal of the game is to write the smallest test method that represents real progress toward your end goal.” - Kent Beck, “Test-Driven Development By Example.”
Uncle Bob once sat with Kent Back and learned how Kent worked: “He would write one line of a failing test and then write the corresponding line of production code to make it pass. Sometimes it was slightly more than one line, but the scale he used was very close to line by line.”
Green
The green stage aims to pass the failing tests quickly. You write the simplest code that fulfills the test requirements. In this stage, don't think about what your code looks like or how efficient it is. The important thing is passing the test.
Refactor
Eliminate all duplication created while getting the test to work. Refactoring involves restructuring and organizing your code. The refactoring stage can happen at any time, whether after one or more red/green cycles.
Refactoring should focus on improving the code's readability and maintainability. Running tests after each refactoring ensures that existing functionality is preserved. Refactoring can also involve tests, but you should avoid refactoring code and tests simultaneously.
Refactoring is not something done at the end, after all the code is written. It is an integral part of the entire TDD process and needs to be done continually.
The Green phase and refactoring should not be confused. First, you need to make all tests green, and then move on to refactoring. Omitting the refactoring stage distorts the essence of the TDD idea.
After completing the refactoring stage, the process enters the “Repeat” phase, which involves cycling back to the “Red” phase to address the next set of requirements or features. Throughout the repeat phase, developers continuously add new tests, refactor existing code, and extend the functionality, all while keeping the codebase reliable and bug-free.
Advantages of Test-Driven Development (TDD)
Test-Driven Development offers numerous benefits that positively impact software development. Let's explore some of the key advantages:
Focus on requirements before writing code
One of the key benefits of test-driven development is that it compels developers to prioritize requirements and design before writing code. By formulating test cases upfront, developers are forced to think through program functionality, potential user scenarios, and desired outcomes. This thoughtful approach leads to a clearer understanding of the project's scope and requirements.
Improved code quality and reduced errors
Since TDD mandates the creation of tests before writing code, the resulting codebase tends to have better test coverage. Comprehensive test suites help catch errors early in the development process, reducing the number of bugs that make it to the production environment. This not only saves time on debugging but also contributes to the overall reliability and stability of the software.
“Case studies were conducted with three development teams at Microsoft and one at IBM that have adopted TDD. The results of the case studies indicate that the pre-release defect density of the four products decreased between 40% and 90% relative to similar projects that did not use the TDD practice.” Microsoft
Another survey found that 92% of developers believe that TDD yields higher quality code, 79% think that TDD promotes simpler design, and 71% consider the approach to be noticeably effective.
Furthermore, TDD enhances code understanding by providing clear examples of expected behavior. Tests can serve as up-to-date documentation, making it easier for developers to comprehend the program's functionality.
Efficient and confident refactoring
During the refactoring phase of TDD, developers can confidently restructure and optimize their code without worrying about introducing defects. The presence of extensive test cases acts as a safety net, ensuring that existing functionality is preserved after code modifications. This makes the refactoring process more efficient and encourages developers to continuously improve the codebase without fear of breaking it.
Improved development speed
Despite conflicting statistics on whether or not TDD directly improves development speed, some evidence suggests that once developers overcome the initial learning curve of the methodology, their development speed tends to increase. This improvement can be attributed to the time saved on subsequent debugging of code and the efficient resolution of bugs during the development process.
“TDD has a learning curve, and while you’re climbing that learning curve, it can and frequently does add 15%-35% to implementation times. But somewhere around the 2-years in mark, something magical started to happen: I started coding faster with unit tests than I ever did without them.” - Eric Elliott, software engineer, editor of JavaScript Scene and The Challenge
Early error detection and cost reduction
Since tests are written before the new code is implemented, TDD allows errors to be detected at an early stage of development. This significantly reduces the likelihood of critical issues surfacing at the final stages or in the finished product, ultimately reducing the cost of development and maintenance.
Greater job satisfaction
TDD had some positive side effects for developers. Knowing that their code is backed by comprehensive tests can boost developers’ confidence in their work and lead to greater job satisfaction.
Limitations of TDD
While TDD offers significant advantages, it's essential to understand its limitations as well. TDD is not a replacement for other types of testing, such as performance, stress, or usability testing. Each testing method serves a unique purpose and complements the overall software quality assurance strategy.
Additionally, the effectiveness and applicability of TDD depend on the individual developer. While TDD might yield excellent results for some developers, others may experience challenges due to their personal preferences, knowledge, and experience. TDD, like any methodology, comes with its own set of requirements, and one of them is that developers need to dedicate time to grasp the principles and practices involved in TDD. It's important to adapt TDD practices based on the specific needs and dynamics of the development team and project.
Consider integrating TDD into your development process
Test-Driven Development offers a disciplined and feedback-driven approach to software development that yields numerous benefits. By prioritizing requirements, fostering better code quality, and enabling efficient refactoring, TDD promotes a reliable and almost bug-free codebase. Early error detection, cost reduction, and code protection during refactoring further contribute to its advantages.
However, TDD is not a one-size-fits-all solution and depends on individual developers' preferences and expertise. While embracing TDD can enhance software development, it should be integrated thoughtfully into the development process, considering the project's unique requirements and team dynamics. Overall, TDD remains a valuable methodology in the pursuit of efficient and high-quality software development.