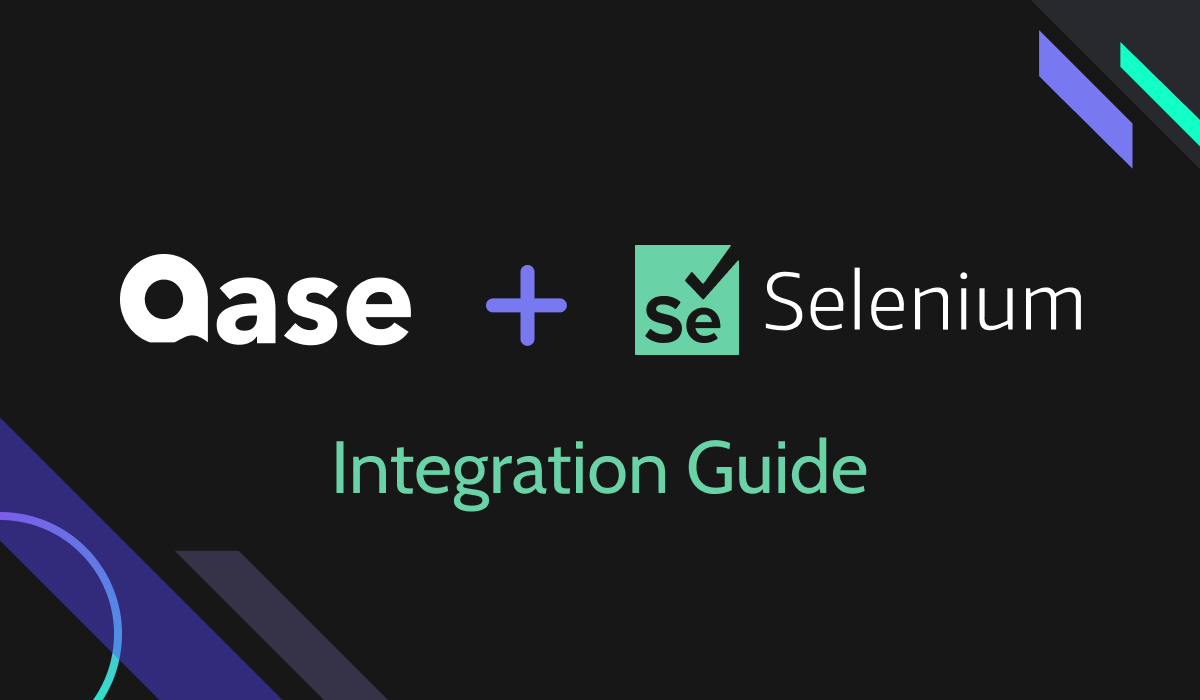
We all know that test automation has become a crucial component in ensuring the quality and reliability of applications. A streamlined testing process not only saves time and resources, but also allows developers to identify and address issues promptly. Integrating powerful test management tools with versatile automation frameworks is essential to accomplishing this.
For those who are new here, Qase is a comprehensive TestOps platform that offers features for managing automated tests and the whole software testing process at scale. Selenium is an open-source automation framework that enables automated testing of web applications across multiple browsers and platforms. By harnessing the power of Python, we bridge the gap between Qase and Selenium to create a test automation pipeline.
In this article, we will guide you step-by-step on how to integrate Qase with Selenium using Pytest. This guide assumes that you have a basic knowledge of Python and Selenium.
Let's get started on the journey to streamline test automation!
Integration with Pytest
Qase provides a RESTful API that can be used to integrate various tool and frameworks like TestNG, JUnit, Cucumber, Playwright, Cypress, Pytest, Robotframework and many others. Check our Github profile for other reporters and integrations.
Why Pytest? Pytest is a commonly used solution for running python tests across the whole software testing pyramid: whether they are end-to-end, integration or unit. It is flexible and allows to create tests with a minimal setup and boilerplate code.
Before we start, we have to prepare a few things:
- Write a simple selenium test
- Install dependencies
- Obtain Qase API token
- Create a new project in Qase to collect results
Selenium test
We will write a very simple test that checks Qase’s website title. You can copy and paste the following code snippet and save it as test_selenium.py
:
import pytest
from selenium import webdriver
def test_title():
driver = webdriver.Chrome()
driver.get('https://qase.io/')
assert driver.title == 'Qase | Test management software for QA teams'
driver.quit()
Dependencies
Now we are ready to setup integration with Qase. All you have to do is to install pytest plugin (reporter) that will handle collection:
pip install qase-pytest
You can verify that reporter has been installed by running this command:
pip show qase-pytest
Check if output is similar to the following:
Name: qase-pytest
Version: 1.0.3
Summary: Qase Pytest Plugin for Qase TestOps and Qase Report
Home-page: https://github.com/qase-tms/qase-python/tree/master/qase-pytest
Author: Qase Team
Author-email: [email protected]
License: apache
Location: /opt/homebrew/lib/python3.11/site-packages
Requires: pytest, qase-python-commons
Required-by:
API Token
To start collecting responses, we have to get API token from Qase. There are two different types: personal token (that can be used on a local machine to run tests) or App Token.
To get personal token, navigate to https://app.qase.io/user/api/token and create a new one:
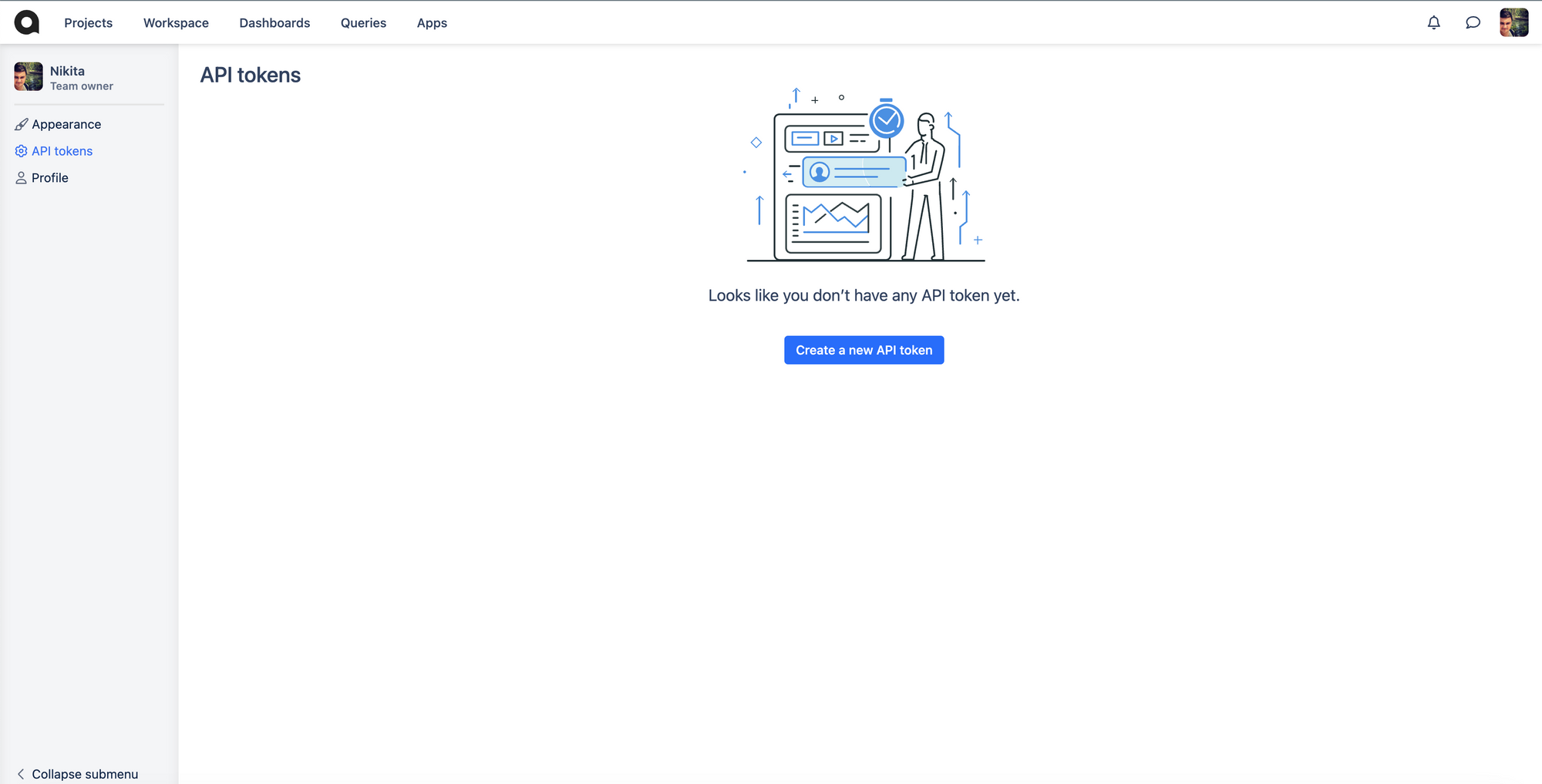
To get App token, go to Apps Marketplace page and choose Pytest from the list:
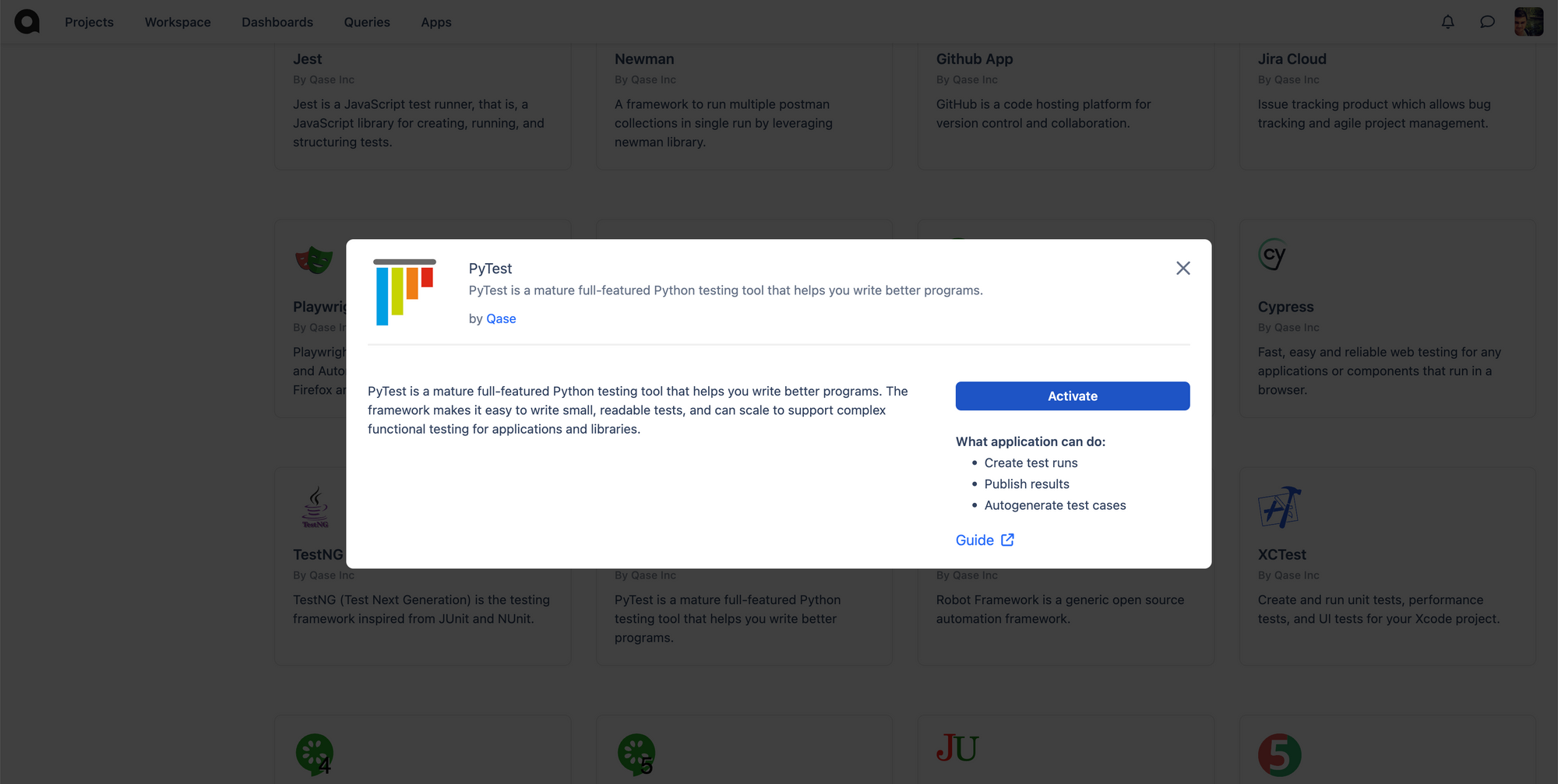
Project
Projects in Qase are containers for all of your tests, runs and defects. Each project can have a different set of custom fields and different workflows. Create a new project that will be used to aggregate results of test execution and save its code.
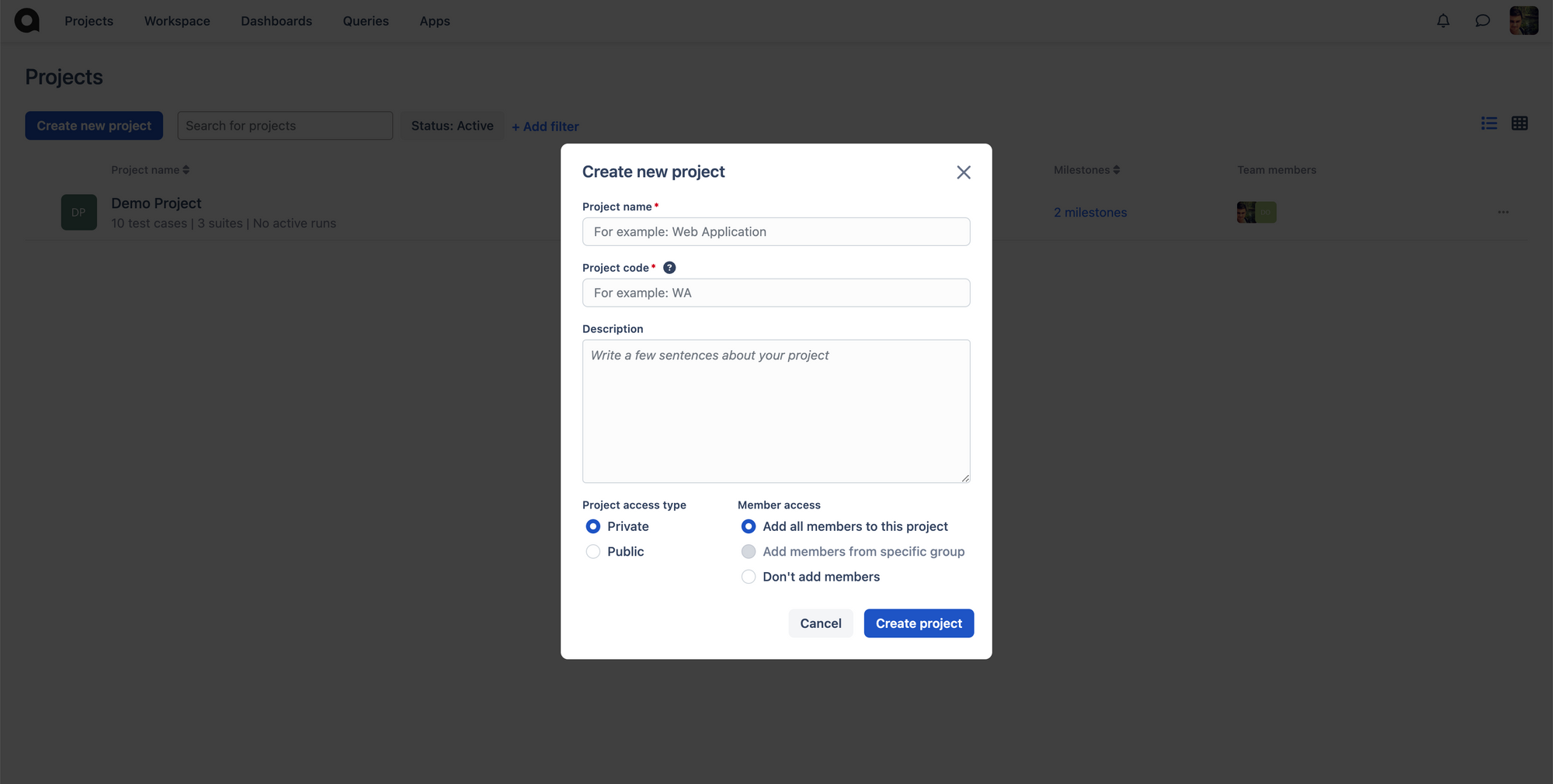
We're ready to go
When we have everything we need in place, we can run our tests. Pass params to:
pytest --qase-mode=testops --qase-to-api-token=<API_TOKEN> --qase-to-project=<PROJECT_CODE>
That’s all.
You should see the results in Qase. What happens under the hood:
- Qase Pytest reporter creates a new run in a selected project.
- It submits the results through the API.
- It creates new test cases in the repository (if you don’t have them).
- It marks a run as completed.
If you don’t want to pass these variables for every execution, you have two options:
- Create an INI file
- Use ENV variables.
To learn more, check our documentation here.
Diving deeper
We have submitted the result of our first selenium test to Qase. But if you are following tests-as-a-code approach, you can use additional methods provided by Qase Pytest Reporter.
Sometimes it's hard to understand the purpose of a test without additional context, that’s why we have prepared context decorators that can make your tests human-readable. Here is an example of the same test from the first part of the article, but with additional decorators:
import pytest
from selenium import webdriver
@qase.title('Selenium test')
@qase.description('This test checks title')
@qase.severity("Blocker")
@qase.layer("e2e")
def test_site_title():
with qase.step("Set up"):
driver = webdriver.Chrome()
with qase.step("Open website"):
driver.get('https://qase.io/')
qase.attach((driver.get_screenshot_as_png(), "image/png", "page.png"))
with qase.step("Check title", "Title should be 'Qase | Test management software for QA teams'")
assert driver.title == 'Qase | Test management software for QA teams'
with qase.step("Tear down"):
driver.quit()
So, what’s going on here:
@qase.title(”string”) | Can be used to define human-readable name of the test |
---|---|
@qase.description(”string”) | Can be used to describe the purpose of test. Markdown is supported. |
@qase.severity(”string”) | Can be used to define test severity. Possible values are defined in project settings. |
@qase.layer(”string”) | Can be used to define test layer (E2E, API, Unit and etc.). Allowed values are defined in project settings. |
qase.attach() | This method is used to attach files to the test. It can be any file: image, video, text and etc. |
@qase.step(”string”, “string”) | This method tells reporter to track a particular step. Using this method you can structure your test in logical groups and track their execution time. This decorator can be applied to a particular method. You can pass step description and expected result as arguments. |
with qase.step(”string”) | Another form of annotating a step, but inside a method. |
Conclusion
Selenium is an industry standard, and mastering it will benefit you. As you can see, it's easy to integrate Qase with Selenium, and this will provide increased efficiency (and ultimately product quality) for your team.
Selenium tests are end-to-end tests, so they are on the top of software testing pyramid. End-to-end tests are slow (compared to integration and unit tests), so it is important to keep their execution time as low as possible. Qase will help you profile your tests and optimize them.